Qualtrics Mouse Tracking
A how-to guide for fast implementation
Over the past few years I have received many requests for direction on how to implement a mouse tracking system for Qualtrics that I wrote a couple years back for a psychology researcher at Virginia Tech. Instead of responding to individual emails, I've decided to post the code publicly along with a short tutorial. Feel free to use the code freely and share with anyone who may be interested.
UPDATE: The code has found many uses, especially and to my knowledge exclusively for researchers. The code has been extended and implemented by Maya B. Mathur and David B. Reichling and is available through Springer: https://link.springer.com/article/10.3758/s13428-019-01258-6
Implementation
As far as I know, Qualtrics does not have a built in way to store & retrieve mouse tracking data. It is, however, quite flexible and allows users to insert javascript and HTML into any question. Using some javascript, the user's mouse position can be recorded at a given frequency, say once every 10ms. Although it is a bit of a hack, we can then use Qualtrics' embedded data variables to store the mouse position and time data. This data can easily be retrieved in Qualtrics using the 'View Results' tab.
There are just three steps to getting things up and running: Create the embedded data variables, insert the mouse tracking code, and configure the 'Next Button'.
Retrieving the Data
Finally we'll want to retrieve the data. The list of x, y positions and time will be stored as strings in the embedded data variables xPos, yPos, and time.
After activating, launching, and completing your survey at least once to get some data, click on the Data Analysis tab and export the data as a .csv file. The results should look like the image below. If the xPos, yPos, and time cells are blank something is wrong.
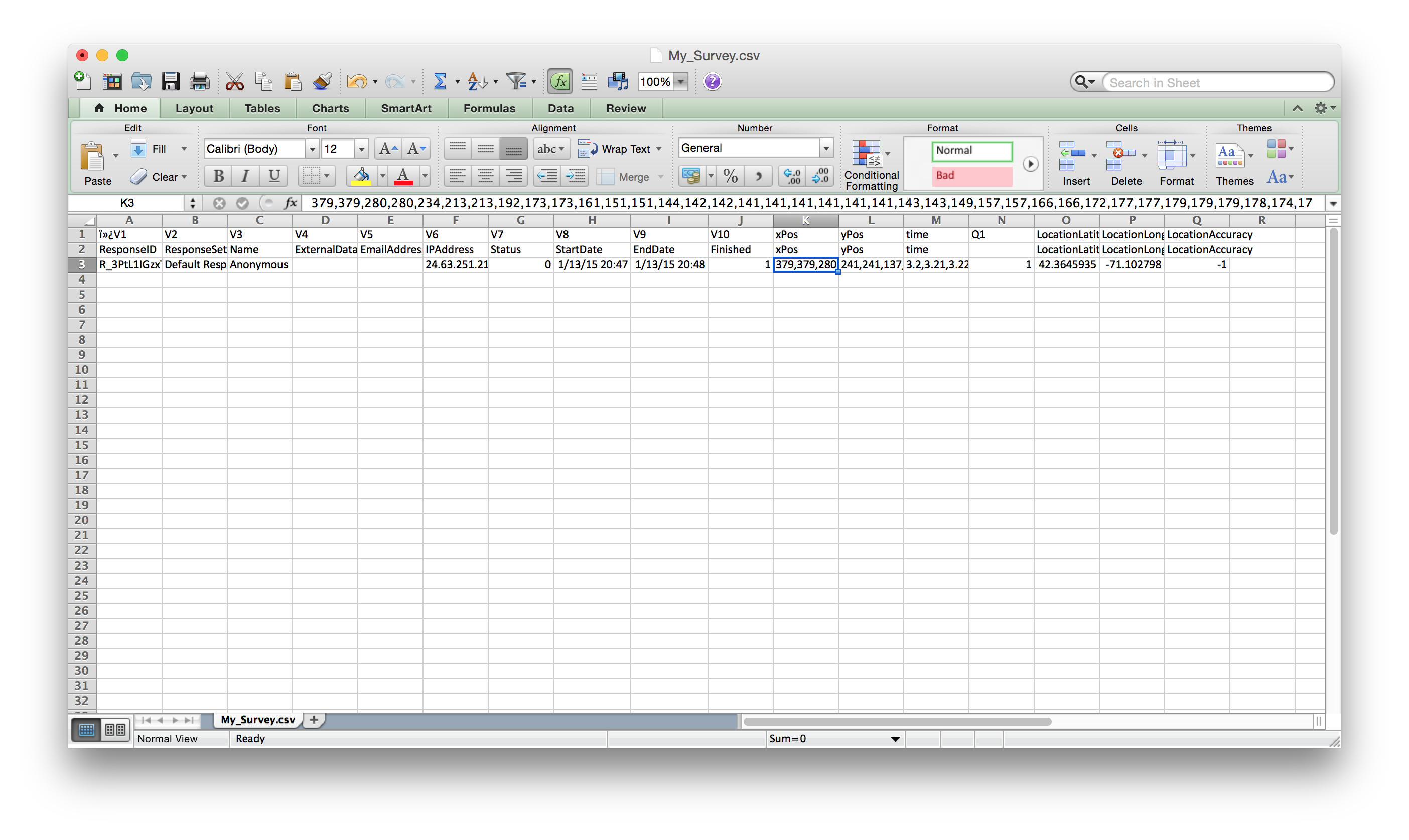
The results will be stored under the xPos, yPos, and time columns, each as a single comma separated string. We want to separate the strings into lists of distinct numbers. To do this, create a separate .csv file and open it in a text editor. Copy and paste each string from the original CSV file as a single line in the new one. You may now save this file and reopen it in Excel or wherever to view or manipulate the data as you desire.
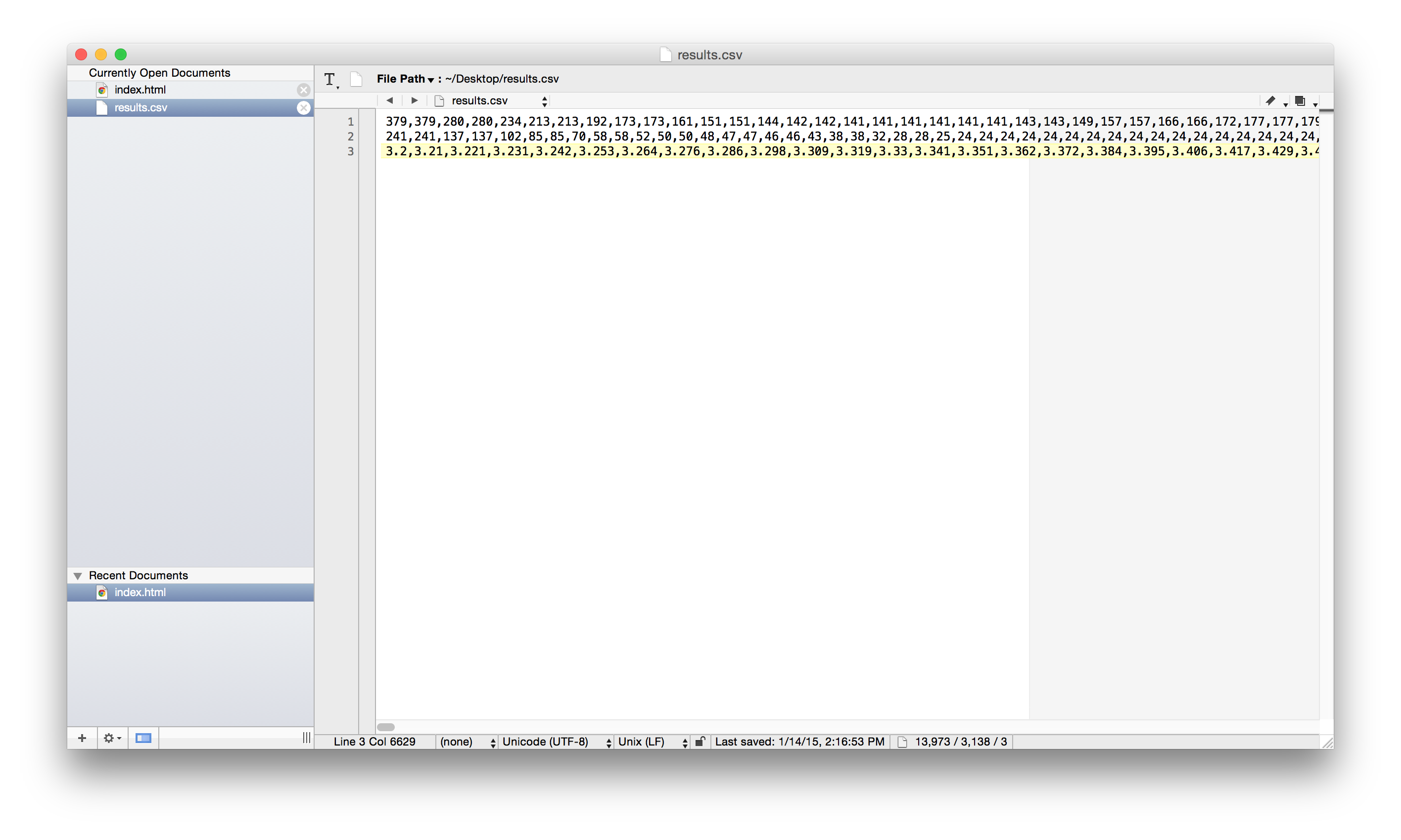
I used Mathematica (simple_process.nb) to do some post processing. Here are some examples of results you can expect:
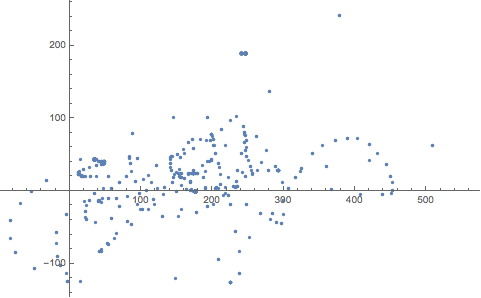
Above is a plot of all (x,y) points for some sample data. Using the time data, I created a short animation that gives a better idea of what is happening:
That should do it! I hope this helps someone out there. Please note that a lot of this could be modified to suit your particular needs - this is just the simplest, most general implementation.